jQuery and the Netflix API – Part 1
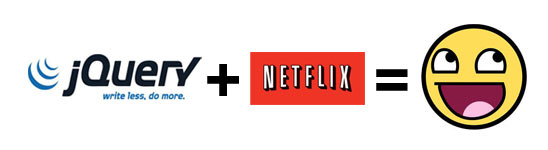
So I finally broke down and signed up for Netflix. My roommate has it and his life seemed too good to be true because of this weekly crimson DVD in the mail. My life has now waned to a driveling mess as I fulfill my movie addiction directly from my living room.
That aside, the first problem I realized was that my roommate and my queue’s were constantly crossing over one another. He would have a DVD out I had next in my queue, or vice versa. Or even worse, we’d have two of the same DVD at the same time!
My solution seemed clear and simple: Use the Netflix API to pull in a feed of both of our feeds, and then parse those feeds with jQeury, only to finally compare them and see where our overlapping tastes lie. Thus, the crossing of the streams would end. Hopefully.
I plan to share my findings along the way, so lets jump into part one of my tutorial on jQuery and the Neflix API.
First, you’ll need to get an account on Mashery for API usage. After that, you’ll need to go through the authentication walkthrough. Netflix walks you through the process, which is extremely tedious, but relatively simple.
Once complete, you’ll need three things to create a feed call and grab your DVD queue:
- user id
- feed token
- consumer key
Your user id and consumer key will be given to you during the authentication process, so they are easy to find. The feed token, however, is a bit more work to get. Head to step 6 of the walkthrough, and run a query for the following URL, getting all of your possible feeds. Replace the XXXXX with your user id:
http://api.netflix.com/users/XXXXX/feeds
The output will include links to all of your feeds, with the feed token on each. Grab the token, and create your atom feed URL like so:
http://api.netflix.com/users/USER_ID/queues/disc?feed_token=FEED_TOKEN&oauth_consumer_key=CONSUMER_KEY&output=atom
Ok, now time for the code. First, lets get some HTML together to dump our feed into. I’ve been playing with HTML5, so we’ll try some of those tags:
HTML
<section id="nfPage"> <header id="branding"> <hgroup> <h1 id="site-title">Netflix Queue</h1> </hgroup> </header> <section id="nfMain"> <ol></ol> </section> <footer id="nfFoot"> <address>Created by <a href="www.stinogle.com">Rob Stinogle</a></address> </footer> </section>
Next, we want to write a simple AJAX request function with jQuery. On complete, we’ll fire off a function to parse the XML:
Javascript
function grabXml() { $.ajax({ type: "GET", //Grab the users queue in atom format url: "http://api.netflix.com/users/USER_ID/queues/disc?feed_token=xxxxxxxxxxxx&oauth_consumer_key=xxxxxxxxxxxx&output=atom", dataType: "xml", //fire off success function success: parseXml }); }
Now we have XML feed. We now need to write the parseXML function to dump that XML into our page:
Javascript
function parseXml(xml) { //find every movie in the queue and print the title $(xml).find("entry").each(function() { //dump the content into the html tags $("#nfMain ol").append("<li>" + $(this).find("title").text() + "</li>"); }); }
And finally, we put it all together, with a few additions, such as our HTML5 doctype:
HTML
<!DOCTYPE html> <html dir="ltr" lang="en-US"> <head> <meta charset="UTF-8" /> <title>Netflix</title> <script type="text/javascript" src="jquery-1.4.2.min.js"></script> <script type="text/javascript"> $(document).ready(function() { grabXml(); }); function grabXml() { $.ajax({ type: "GET", //Grab the users queue in atom format url: "http://api.netflix.com/users/USER_ID/queues/disc?feed_token=xxxxxxxxxxxx&oauth_consumer_key=xxxxxxxxxxxx&output=atom", dataType: "xml", //fire off success function success: parseXml }); } function parseXml(xml) { //find every movie in the queue and print the title $(xml).find("entry").each(function() { //dump the content into the html tags $("#nfMain ol").append("<li>" + $(this).find("title").text() + "</li>"); }); } </script> </head> <body> <section id="nfPage"> <header id="branding"> <hgroup> <h1 id="site-title">Netflix Queue</h1> </hgroup> </header> <section id="nfMain"> <ol></ol> </section> <footer id="nfFoot"> <address>Created by <a href="www.stinogle.com">Rob Stinogle</a></address> </footer> </section> </body> </html>
And that concludes part one of my first real in depth tutorial on this blog. If you made it this far, then you are a saint. Feel free to ask any questions you have! Part two will be coming soon; we’ll add another feed, and start to compare the two queues with javascript.
Leave a Reply